linear github integration | Create Issues from Code Commits using Autogen
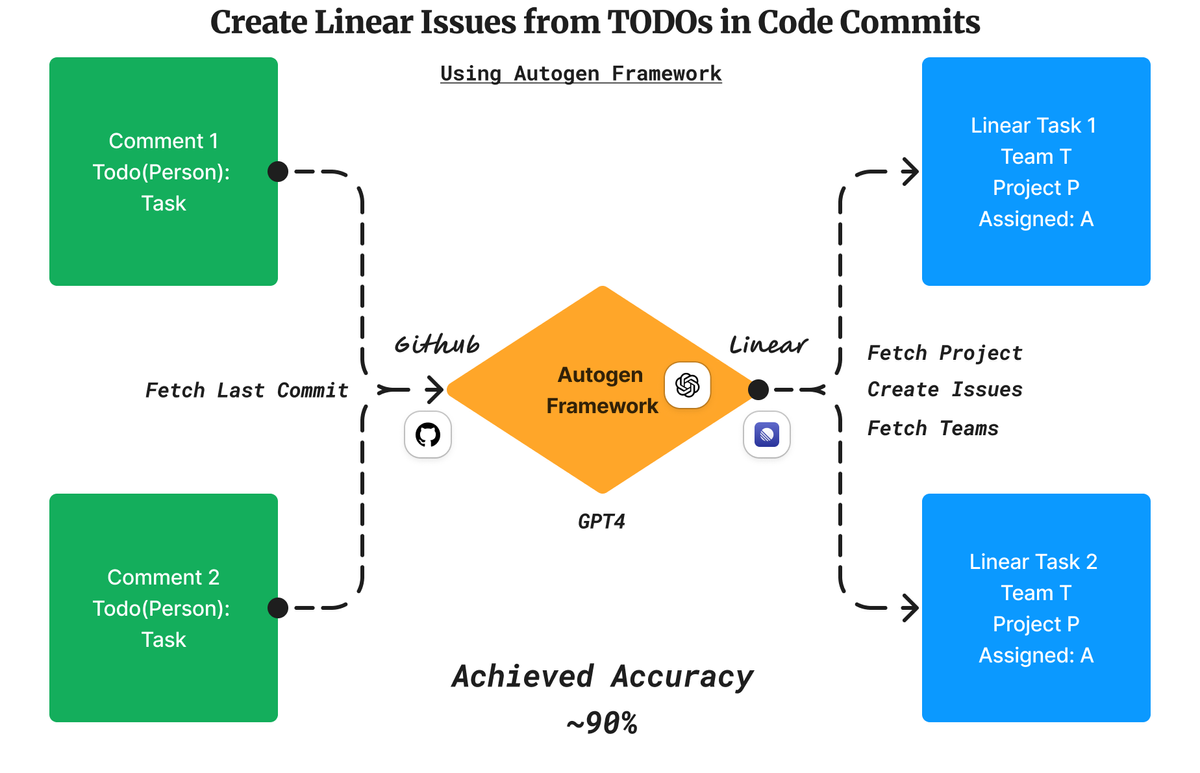
Introduction to Linear GitHub Integration
I use #TODO
comments in commit messages to flag future tasks. Tracking these tasks manually is inefficient and error-prone.
I am trying to build using Autogen Agents - Convert these TODOs from Github code commits into Linear tasks with github linear integration.
Background - Integrate Composio Agent to Linear and GitHub
Autogen Agentic Framework allows for natural language understanding and action-taking and is super easy to start with.
Composio is a very easy way to connect your Autogen Agents with real life tools. We will be using it to integrate our agent to Linear and Github.
TL;DR: Show me the Code
Setup
!pip install pyautogen composio_autogen --quiet
Installing the relevant packages
Initialising Agents for GitHub Linear Integration
To initialise, perform the following steps:
- Configure LLM: Use the
gpt-4-1106-preview
model. Provide the OpenAI environment key through an environment variable or modify the code directly. - Set Up Assistant Agent: Create it with a system prompt and the LLM configuration. Adjust as needed to improve outcomes.
- Establish User Proxy Agent: These agents simulate users, interacting with Autogen agents. They include a function to end the process upon detecting the word "Terminate," as specified in the system prompt.
import os
from autogen import AssistantAgent, UserProxyAgent
from composio_autogen import App, Action, ComposioToolset
llm_config = {
"config_list": [
{
"model": "gpt-4-1106-preview",
"api_key": os.environ.get(
"OPENAI_API_KEY", "sk-123131****"
),
}
]
}
super_agent = AssistantAgent(
"chatbot",
system_message="""You are a super intelligent personal assistant.
You have been given a set of tools that you are supposed to choose from.
You decide the right tool and execute it to achieve your task.
Reply TERMINATE when the task is done or when user's content is empty""",
llm_config=llm_config,
)
# create a UserProxyAgent instance named "user_proxy"
user_proxy = UserProxyAgent(
"user_proxy",
is_termination_msg=lambda x: x.get("content", "")
and "TERMINATE" in x.get("content", ""),
human_input_mode="NEVER", # Don't take input from User
code_execution_config={"use_docker": False},
)
Agent Initialisation Code
Connect GitHub and Linear using Composio
We need to connect Github and linear to our agents and via function calling they ideally accomplish the task. I will be using Composio
to do that.
- Authorise
Github
andLinear
connections, so Autogen Agents can interact with it.
pip install composio-autogen
composio-cli add github # Authorise Github
composio-cli add linear # Authorise Linear
Adding Github and Linear Connections to Composio. (Run on Terminal)
- Register the tools with Autogen Agent
# Initialise the Composio Tool Set
composio_tools = ComposioToolset()
# Register the authorised Applications, with our agent.
composio_tools.register_tools(
tools=[App.LINEAR, App.GITHUB], caller=super_agent, executor=user_proxy
)
This allows our agent and user proxy to interact with external Applications
Executing the Task
Define the task so agent can execute it. 🚀
task = """For all the todos in my last commit of SamparkAI/Docs,
create a linear issue on project name hermes board and assign to right person"""
response = user_proxy.initiate_chat(super_agent, message=task)
print(response.chat_history)
And it works!
The issues have been successfully created on the Linear board for the project "Hermes" as follows:
1. **Extracted TODO from Docs commit**
- Issue ID: `d578a113-a7ab-4084-9a76-5dbbd4f23b21`
- Description: A new Linear issue created for the TODO from the latest commit in the SamparkAI/Docs repository.
2. **Evaluate documentation structure**
- Issue ID: `0c710f8a-8037-4bf4-8ebd-a801531117b5`
- Description: Evaluate and update the documentation structure based on the latest standards.
3. **Review and finalize API docs**
- Issue ID: `961651df-ad3c-4b4c-b162-931eead8be19`
- Description: Review the API documentation to ensure accuracy and completeness.
All issues have been assigned to the appropriate team member. The task is now complete.
Terminate
Output From Autogen
Final Code
#!pip install pyautogen composio_autogen --quiet
from autogen import AssistantAgent, UserProxyAgent
from composio_autogen import App, Action, ComposioToolset
import os
llm_config = {
"config_list": [
{
"model": "gpt-4-turbo-preview",
"api_key": os.environ.get("OPENAI_API_KEY", "sk-123***"),
}
]
}
super_agent = AssistantAgent(
"chatbot",
system_message="""You are a super intelligent personal assistant.
You have been given a set of tools that you are supposed to choose from.
You decide the right tool and execute it to achieve your task.
Reply TERMINATE when the task is done or when user's content is empty""",
llm_config=llm_config,
)
# create a UserProxyAgent instance named "user_proxy"
user_proxy = UserProxyAgent(
"user_proxy",
is_termination_msg=lambda x: x.get("content", "")
and "TERMINATE" in x.get("content", ""),
human_input_mode="NEVER", # Don't take input from User
code_execution_config={"use_docker": False},
)
# Initialise the Composio Tool Set
composio_tools = ComposioToolset()
# Register the preferred Applications, with right executor.
composio_tools.register_tools(
tools=[App.LINEAR, App.GITHUB], caller=super_agent, executor=user_proxy
)
task = """For all the todos in my last commit of SamparkAI/Docs,
create a linear issue on project name hermes board and assign to right person"""
response = user_proxy.initiate_chat(super_agent, message=task)
print(response.chat_history)
Complete Code
Join our Discord Community and check out what we're building!